5. Dependency Inversion Principle
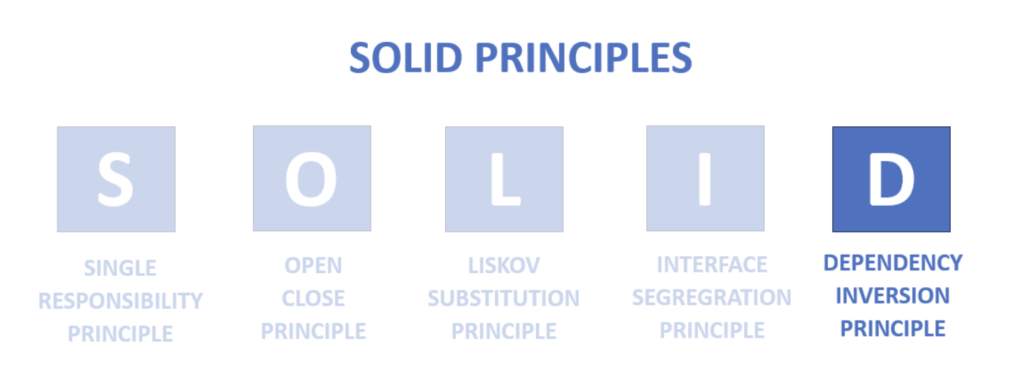
Introduction
In software development, managing dependencies effectively is crucial for creating scalable and maintainable systems. The Dependency Inversion Principle (DIP) is a fundamental design guideline under the SOLID principles that helps achieve this by recommending high-level modules should not depend on low-level modules but both should depend on abstractions. By following DIP, software systems can reduce tight coupling, thereby enhancing their flexibility and robustness.
Understanding the Dependency Inversion Principle
DIP focuses on decoupling software modules, ensuring that both high-level business logic and low-level implementation details rely on abstractions rather than concrete implementations. The mantra of DIP is –
Depend on abstractions, not on concretions.
This strategy promotes easier maintenance, better scalability, and enhanced adaptability to changes.
Why is DIP Important?
- Enhances Modularity: Dependence on abstractions rather than concrete implementations allows software modules to be more interchangeable and updated more easily.
- Increases Flexibility: Changes to the implementation of a module do not force modifications on other modules that use it.
- Reduces Dependencies: Direct dependencies among components are minimized, simplifying upgrades and maintenance.
DIP in Action:
1. Repository Management – Java Example
Consider an application that fetches data using different types of databases.
Without DIP:
class MySQLRepository {
public Data fetchData() {
// Fetch data from MySQL database
}
}
class PostgreSQLRepository {
public Data fetchData() {
// Fetch data from PostgreSQL database
}
}
class Service {
private MySQLRepository repository = new MySQLRepository();
// Switching to PostgreSQL requires changes to the Service class.
public Data performAction() {
return repository.fetchData();
}
}
Impact of Violation:
- Code Rigidity: Changing from
MySQLRepository
toPostgreSQLRepository
necessitates changes to theService
class, demonstrating inflexibility and tight coupling.
With DIP:
interface Repository {
Data fetchData();
}
class MySQLRepository implements Repository {
public Data fetchData() {
// Fetch data from MySQL database
}
}
class PostgreSQLRepository implements Repository {
public Data fetchData() {
// Fetch data from PostgreSQL database
}
}
class Service {
private Repository repository;
public Service(Repository repository) {
this.repository = repository;
}
public Data performAction() {
return repository.fetchData();
}
}
Impact of Adhering to DIP:
- Flexibility and Scalability: The
Service
class can seamlessly switch between different database implementations without modifications, thanks to its dependency on theRepository
interface.
2. Notification System – Python Example
Let’s consider a notification system that can send messages via different services.
Without DIP:
class EmailService:
def send_message(self, message):
print(f"Sending email: {message}")
class SMSService:
def send_message(self, message):
print(f"Sending SMS: {message}")
class NotificationService:
def __init__(self):
self.email_service = EmailService();
// Switching to SMS service requires changes in NotificationService.
def notify(self, message):
self.email_service.send_message(message)
}
Impact of Violation:
- Reduced Modularity: Changing the messaging method involves modifications in the
NotificationService
, demonstrating a high degree of coupling.
With DIP:
class MessageService:
def send_message(self, message):
pass
class EmailService(MessageService):
def send_message(self, message):
print(f"Sending email: {message}")
class SMSService(MessageService):
def send_message(self, message):
print(f"Sending SMS: {message}")
class NotificationService:
def __init__(self, service: MessageService):
self.service = service
def notify(self, message):
self.service.send_message(message)
}
Impact of Adhering to DIP:
- High Adaptability:
NotificationService
is designed to work with any service that implements theMessageService
interface, allowing for easy integration of new messaging types without internal changes.
Conclusion
The Dependency Inversion Principle encourages designing software where both high-level and low-level modules depend on shared abstractions, rather than on concrete details. This approach not only simplifies maintenance but also makes the software robust against changes and easier to scale.
Now that you know about this principle, let us know how you can apply DIP and change the way you currently structure your software. Consider examples from your work where dependency inversion could enhance flexibility and testability. Share your thoughts and experiences in the comments below.