3. Liskov Substitution Principle
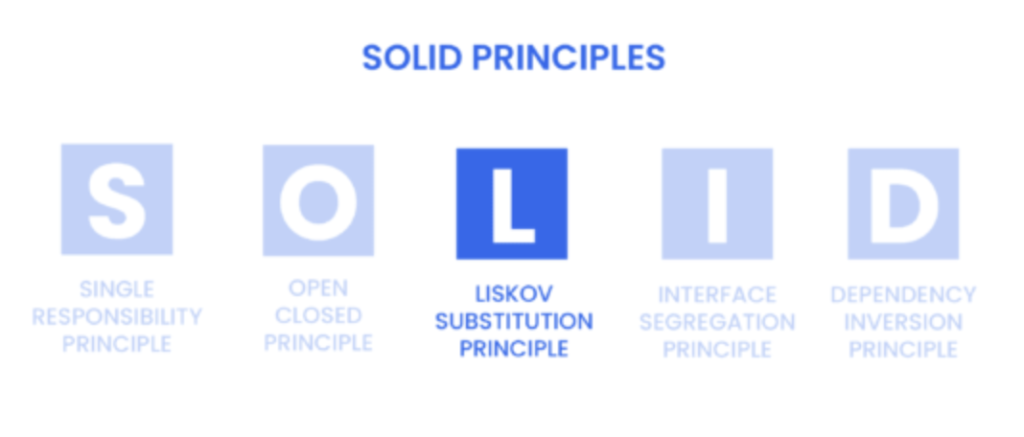
Introduction
When building software systems, ensuring that components are interchangeable without causing errors is crucial for robust architecture. The Liskov Substitution Principle (LSP), a key element of the SOLID principles, asserts that objects of a superclass should be replaceable with objects of its subclasses without affecting the application’s correctness. This principle promotes reliability and reusability in object-oriented programming.
Understanding the Liskov Substitution Principle
LSP is designed to ensure that a subclass can stand in for its superclass without disrupting the functionality of the program. Adhering to this principle helps in building software that is easy to upgrade and maintain, with components that are interchangeable. In simple terms, it is –
A subclass should fit perfectly in place of its parent class without causing any issues.
Why is LSP Important?
- Enhances Modularity: LSP makes it easier to manage and evolve software systems as new types of components can replace existing ones without additional modifications.
- Reduces Bugs: By ensuring that subclasses can serve as stand-ins for their superclasses, LSP reduces the likelihood of errors during code extension.
- Improves Code Flexibility: It allows developers to use polymorphism more effectively, making the software easier to understand and modify.
LSP in Action: Java Example
Consider a class hierarchy where Bird
is a superclass, and it has several subclasses including Duck
and Ostrich
.
Without LSP:
class Bird {
void fly() {
// logic to fly
}
}
class Duck extends Bird {
// Ducks can fly
}
class Ostrich extends Bird {
void fly() {
throw new UnsupportedOperationException("Ostriches cannot fly");
}
}
In this scenario, using an Ostrich
object in place of a Bird
can cause the program to fail if the fly
method is called.
With LSP:
abstract class Bird {
}
abstract class FlyingBird extends Bird {
void fly() {
// logic to fly
}
}
class Duck extends FlyingBird {
// Ducks can fly
}
class Ostrich extends Bird {
// No fly method
}
This design adheres to LSP by separating birds that can fly from those that cannot, eliminating the issue of inappropriate method calls.
LSP in Action: Python Example
Let’s look at a payment system where Payment
is a superclass, and it has several subclasses such as CreditCardPayment
and CashPayment
.
Without LSP:
class Payment:
def process_payment(self, amount):
pass
class CreditCardPayment(Payment):
def process_payment(self, amount):
print("Processing credit card payment")
class CashPayment(Payment):
def process_payment(self, amount):
raise NotImplementedError("Cash payments are not supported")
Using CashPayment
in a context expecting a Payment
can lead to runtime errors due to unsupported operations.
With LSP:
class Payment:
def process_payment(self, amount):
pass
class CreditCardPayment(Payment):
def process_payment(self, amount):
print("Processing credit card payment")
class CashPayment(Payment):
def process_payment(self, amount):
print("Processing cash payment")
By ensuring all subclasses can indeed perform process_payment
, we maintain the integrity of the system.
Conclusion
The Liskov Substitution Principle is fundamental in creating scalable and robust software architectures. By ensuring that subclasses can effectively replace their superclasses, developers can build systems that are easier to maintain and extend without fear of breaking existing functionality.
Now that you know about LSP, think about how it might be applied in your current projects and reflect on any past issues where violating LSP caused problems.