1. Single Responsibility Principle
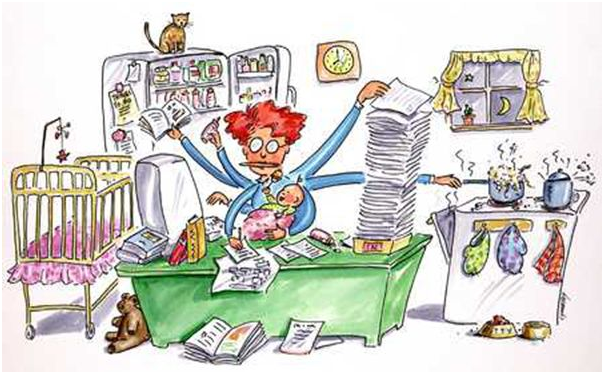
Introduction
Just as humans can perform multiple tasks but often achieve better results by focusing on one task at a time, softwarre components are most effective when they concentrate on single responsibility too! This approach ensures higher quality and better performance. Let’s explore the Single Responsibility Principle in action, examine how applying this principle to software classes and functions to enhance the code clarity and maintainability.
Understanding Single Responsibility Principle
The Single Responsibility Principle simplifies the development process by limiting the impact of changes. By ensuring that a class has only one responsibility, we isolate changes to just one part of the codebase, making it easier to understand, test, and maintain.
Why is SRP Important?
-
- Easier to Modify: Classes with a single responsibility have fewer test cases, making them less susceptible to bugs when changes are made.
-
- Enhanced Modularity: SRP leads to more modular code, making it easier to reuse classes.
-
- Simplified Debugging and Testing: With classes handling just one functionality, identifying and fixing bugs becomes much simpler.
SRP in Action:
Let’s see the Single Responsibility Principle in action through concrete examples. We’ll explore how adhering to SRP can transform cluttered code into clear, modular components in both Java and Python, demonstrating the practical benefits of this principle.
Java Example about Employee Management System –
Consider an application that manages employee information. Let’s say we have a class that handles both the storage and the display of employee data. According to SRP, these two tasks should be separated.
public class Employee {
private String name;
private int age;
public void saveEmployeeToDatabase() {
// Logic to save employee data to a database
}
public void displayEmployeeDetails() {
// Logic to display employee details on a user interface
}
}
Impact of Violation:
-
- Coupling Between Different Functionalities: The
Employee
class is responsible for both data persistence and data presentation. Changes in the database schema or the user interface layout would require modifications to the same class, which increases the risk of introducing bugs affecting unrelated functionalities.
- Coupling Between Different Functionalities: The
-
- Difficulty in Scaling: If the application needs to support different ways of displaying or storing employee data (e.g., saving to a different database or displaying on a different platform), the class would become even more complex and harder to manage.
-
- Challenges in Maintenance and Testing: Testing this class would be cumbersome as tests need to cover both database interactions and user interface rendering. This makes the tests more complex and less focused.
Now lets see how the code looks like while adhering to SRP:
public class Employee {
private String name;
private int age;
}
public class EmployeeRepository {
public void saveEmployee(Employee employee) {
// Logic to save employee data to a database
}
}
public class EmployeeDisplay {
public void displayEmployeeDetails(Employee employee) {
// Logic to display employee details on a user interface
}
}
Python Example for Logging System –
Let’s apply SRP to a simple logging system. Initially, a class might handle both the tasks of logging messages to a console and to a file.
class Logger:
def log(self, message):
print(f"Log to console: {message}")
with open("logfile.txt", "a") as file:
file.write(message + "\n")
Impact of Violation:
-
- Mixing Output Channels: The
Logger
class handles both console output and file writing within the same method. This coupling means that any changes to the logging format or method for one output could inadvertently affect the other.
- Mixing Output Channels: The
-
- Complicated Configuration and Error Handling: If logging to the file fails (e.g., the file is not writable), it could potentially impact the console logging as well, especially if not handled properly.
-
- Harder to Extend: Suppose you later decide to add additional logging outputs, such as to a network server or a cloud-based logging service. In that case, the class will grow even more complex, violating SRP further and making the system harder to extend and maintain.
Now lets see how this example looks like while adhering to SRP:
class ConsoleLogger:
def log(self, message):
print(f"Log to console: {message}")
class FileLogger:
def log(self, message):
with open("logfile.txt", "a") as file:
file.write(message + "\n")
Do you see the difference? It is much cleaner and modular! And, if you want to make any changes to say ConsoleLogger
implementation, it wouldnt impact the FileLogger
functionality.
Conclusion:
Many developers mistakenly think the Single Responsibility Principle means that a class should only do one thing. However, this principle should apply more broadly. It’s not just about classes; every function you write should also focus on performing only one task. Think of it this way: every piece of your code, whether it’s a class or a function, should have just one reason to change. This approach helps keep each component simple and focused, making your code easier to manage and update.
Implementing the Single Responsibility Principle is all about understanding the importance of creating a sustainable and easily adaptable codebase. As we’ve seen with our Java and Python examples, adhering to SRP not only simplifies the development process but also enhances the overall architecture of applications.