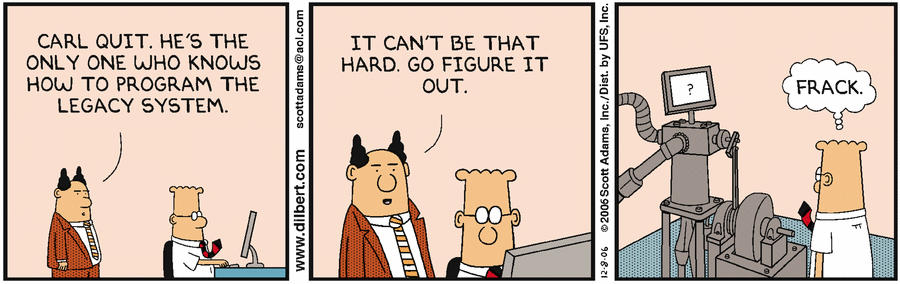
Introduction
Lets visualise a scenario where Carl, the only developer who knows how to program a critical legacy system, decides to quit. Suddenly, the team is left in a mess, not knowing how to manage or update the system. This predicament highlights a common pitfall in software development: over-reliance on specific individuals for knowledge and maintenance of a system. It underscores the importance of designing software that is resilient and adaptable, principles that are central to the Open/Closed Principle (OCP). OCP advocates for software entities to be open for extension but closed for modification, enabling systems to evolve without the need for extensive reworking or specialized knowledge. Let’s explore how applying OCP can transform a software system into a more flexible, maintainable, and scalable architecture.
Understanding the Open/Closed Principle
Software entities like classes, functions, modules, interfaces, etc. should be open for extension, but remain closed for modification.
– Open/Closed Principle
OCP is a fundamental design guideline that encourages developers to write code that doesn’t have to be changed every time the requirements change. Instead, developers should be able to extend existing code to introduce new functionality. This approach reduces the risk of bugs because you’re not modifying the existing tested and proven code.
Why is OCP Important?
OCP in Action:
Java Example – Report Generation System
Imagine a report generation system where we initially only needed to generate HTML reports, but now we also need to support PDF reports.
Without OCP:
class ReportGenerator {
public void generateReport(String reportType) {
if (reportType.equals("HTML")) {
// Generate HTML report
} else if (reportType.equals("PDF")) {
// Generate PDF report
}
}
}
Impact of Violation:
ReportGenerator
class must be modified. This can introduce bugs in the existing report generation logic due to changes in a class that already works correctly for current report types.With OCP:
interface ReportGenerator {
void generateReport();
}class HtmlReportGenerator implements ReportGenerator {
public void generateReport() {
// Generate HTML report
}
}
class PdfReportGenerator implements ReportGenerator {
public void generateReport() {
// Generate PDF report
}
}
With OCP, we can see that new report types can be added without modifying existing code, ensuring ease of extending functionality with minimal errors.
Python Example – Graphic Rendering System
Let’s consider a simple graphic rendering system where we might start with rendering shapes, but later need to add filters.
Without OCP:
class GraphicRenderer:
def render(self, shape):
if shape.type == 'circle':
# Render a circle
elif shape.type == 'square':
# Render a square
# Adding a new shape would require changing the GraphicRenderer class.
Impact of OCP Violation:
GraphicRenderer
class is directly dependent on specific shapes. Adding a new shape means modifying this class, increasing the risk of errors in existing rendering functionality.With OCP:
class Shape:
def render(self):
pass
class Circle(Shape):
def render(self):
# Render a circle
class Square(Shape):
def render(self):
# Render a square
}# you can add new shapes by creating a class for that shape and extending the Shape class
With OCP, we can see that new shapes can be added by simply extending the Shape
class, ensuring stability and scalability.
Conclusion
The Open/Closed Principle is about building software systems that accommodate growth and change as naturally as possible. By adhering to OCP, developers can extend the capabilities of their software without the constant risk of breaking existing functionality.
Can you now reflect on your own projects? Are there areas where applying OCP could simplify the addition of new features?